In this post, we will learn how to add a key to a React Fragment. Fragments are useful for grouping elements without adding extra nodes to the DOM. While using them with the concise syntax <> </>
is common, adding a key prop becomes tricky.
The Traditional Syntax for Adding a Key
To add a key to a React fragment, we’ll use the traditional syntax:
<React.Fragment key={uniqueKey}>
{/* item content here */}
</React.Fragment>
This syntax allows us to achieve the same result of grouping elements without introducing additional DOM nodes.
Let’s look at a practical example:
import React from "react";
import "./App.css";
const App = () => {
const items = ["Apple", "Banana", "Cherry", "Date", "Elderberry"];
return (
<div className="container">
{items.map((item, index) => (
<React.Fragment key={index}>
<p className="item-text">{item}</p>
</React.Fragment>
))}
</div>
);
};
export default App;
Here, we’ve used the traditional syntax for React Fragments to add a key prop. This approach allows us to group elements in a list without adding extra DOM nodes.
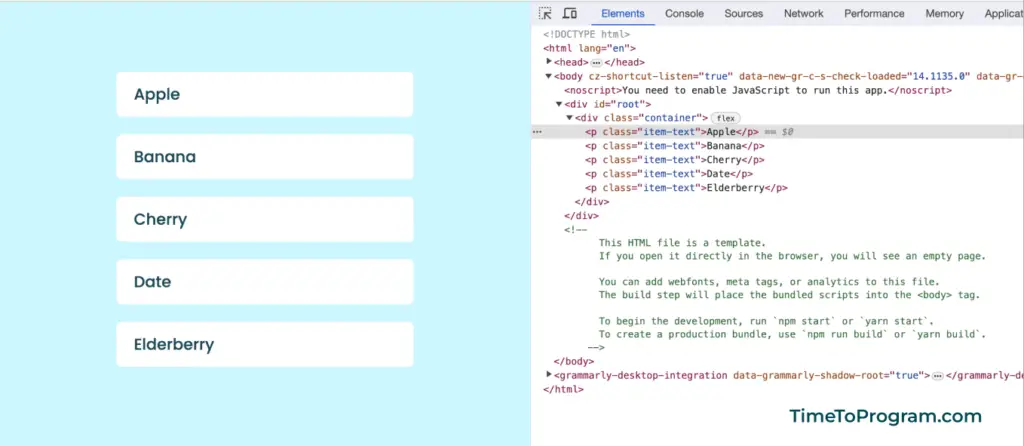
Using the Fragment Element
If we prefer a more concise approach, we can import the Fragment
element from React:
import React, { Fragment } from "react";
import "./App.css";
const App = () => {
const items = ["Apple", "Banana", "Cherry", "Date", "Elderberry"];
return (
<div className="container">
{items.map((item, index) => (
<Fragment key={index}>
<p className="item-text">{item}</p>
</Fragment>
))}
</div>
);
};
export default App;
Using Fragment
from the React package achieves the same result but with a more concise import statement.
When adding keys to React fragments, remember these best practices:
- Each key within a list of fragments must be unique. Repeating keys will trigger an “Encountered two children with the same key” warning.
- Avoid attempting to access the
key
property as a prop within your components. Doing so will result in an “undefined” warning sincekey
is a reserved property for React’s internal use.
Conclusion
In conclusion, adding a key
to a React fragment is a crucial step for optimizing our React app. Whether we opt for the traditional or concise syntax, understanding key prop best practices will help us build robust, high-performance React components.