Today in this post, let us look at how to change the background color of the selected item in the recyclerview.
All other list items background color will be changed back to the default color when the user selects an item.
Change Background Color of Selected Item in RecyclerView
Firstly, I have created a new android project and then in the activity_main.xml, I have added the recyclerview.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
</androidx.constraintlayout.widget.ConstraintLayout>
Now let’s create a new layout resource file recyclerview_list_item.xml for our list item. In this file, we have a simple cardview and a textview within a linear layout.
recyclerview_list_item.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.cardview.widget.CardView xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:cardCornerRadius="8dp"
android:layout_margin="8dp"
android:elevation="0dp"
xmlns:app="http://schemas.android.com/apk/res-auto">
<LinearLayout
android:id="@+id/cardView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="20dp">
<TextView
android:id="@+id/textView_listItem"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="18sp"
android:letterSpacing="0.1"
android:textColor="@color/white"/>
</LinearLayout>
</androidx.cardview.widget.CardView>
Write a recyclerview adapter RecyclerviewAdapter.kt.
RecyclerviewAdapter.kt
package com.timetoprogram.recyclerviewexample
import android.graphics.Color
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.widget.LinearLayout
import android.widget.TextView
import androidx.recyclerview.widget.RecyclerView
import kotlinx.android.synthetic.main.recyclerview_list_item.view.*
class RecyclerviewAdapter(private val listItems: ArrayList<String>): RecyclerView.Adapter<RecyclerviewAdapter.ViewHolder>() {
private var selectedItemPosition: Int = 0
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ViewHolder {
val view = LayoutInflater.from(parent.context).inflate(R.layout.recyclerview_list_item, parent, false)
return ViewHolder(view)
}
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
holder.itemLabel.text = listItems[position]
holder.itemView.setOnClickListener {
selectedItemPosition = position
notifyDataSetChanged()
}
if(selectedItemPosition == position)
holder.cardView.setBackgroundColor(Color.parseColor("#DC746C"))
else
holder.cardView.setBackgroundColor(Color.parseColor("#E49B83"))
}
override fun getItemCount(): Int {
return listItems.size
}
class ViewHolder(itemView: View): RecyclerView.ViewHolder(itemView){
val itemLabel: TextView = itemView.textView_listItem
val cardView: LinearLayout = itemView.cardView
}
}
In the above code within onBindViewHolder, we have defined the on-click listener for the list item. when the user selected an item the background color of that item will be changed. Other list items background color will be changed back to the default color.
Here I have set the selectedItemPosition variable value to 0. It makes the first item of the list selected by default. You can change the selectedItemPosition value to -1 so that no item will be selected until the user selects one.
Also check out:
In the MainActivity.kt, I have set the layout manager and adapter to the recycler view.
MainActivity.kt
package com.timetoprogram.recyclerviewexample
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import androidx.recyclerview.widget.LinearLayoutManager
import androidx.recyclerview.widget.RecyclerView
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val itemList = ArrayList<String>()
for (i in 0..10){
itemList.add("List Item $i")
}
val recyclerView = findViewById<RecyclerView>(R.id.recyclerView)
recyclerView.layoutManager = LinearLayoutManager(this)
recyclerView.adapter = RecyclerviewAdapter(itemList)
}
}
Output:
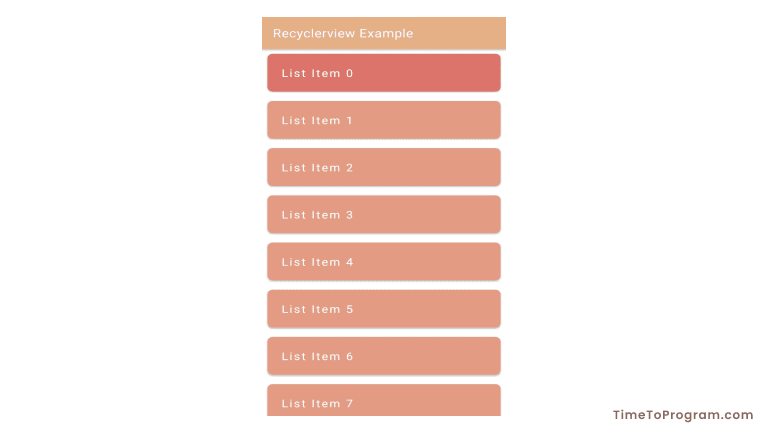