In today’s blog post, we are going to learn how to create a resend OTP countdown timer in React JS using React hooks. In this tutorial, we will implement a 1-minute and 30 seconds countdown timer.
By implementing this countdown timer in the OTP screen we are making the user wait before requesting a new OTP message. And by this, we may also reduce some redundant API calls.
Steps to implement OTP Countdown Timer
- Creating the UI
- Implementing the Timer
- Handling Resend OTP
Creating the UI
App.js
function App() {
return (
<div className="container">
<div className="card">
<h4>Verify OTP</h4>
<input placeholder="Enter OTP" />
<div className="countdown-text">
<p>Time Remaining: 01:25</p>
<button style={{ color: "#FF5630" }}>Resend OTP</button>
</div>
<button className="submit-btn">SUBMIT</button>
</div>
</div>
);
}
export default App;
In the above code, we have written the JSX code for our OTP screen. Which contains an input element to get the OTP, a resend OTP button, and a submit button. Now our UI looks like this.

Implementing the Timer
Now let’s define some useState variables to hold the OTP, minutes, and seconds value.
const [otp, setOtp] = useState("");
const [minutes, setMinutes] = useState(1);
const [seconds, setSeconds] = useState(30);
In the above code, we have set the minutes state variable to 1 and the seconds state variable to 30.
For implementing the countdown timer, we will use the setInterval() method which will allow us to call a function at a specified time interval. Here is the code for the countdown timer written inside the useEffect hook.
useEffect(() => {
const interval = setInterval(() => {
if (seconds > 0) {
setSeconds(seconds - 1);
}
if (seconds === 0) {
if (minutes === 0) {
clearInterval(interval);
} else {
setSeconds(59);
setMinutes(minutes - 1);
}
}
}, 1000);
return () => {
clearInterval(interval);
};
}, [seconds]);
We have set passed 1000 milliseconds as a delay to the setInterval() method so that our function will be invoked with a one-second delay between each call.
Inside setInterval, we are subtracting the ‘seconds’ state variable by one if it is greater than zero. If seconds and minutes are equal to zero we are stopping the timer using the clearInterval() method.
If the minutes state variable is not equal to zero we are updating seconds to 59 and decrementing minutes by one.
Then we will display the ‘minutes’ and ‘seconds’ inside our JSX code.
<div className="container">
<div className="card">
<h4>Verify OTP</h4>
<input
placeholder="Enter OTP"
value={otp}
onChange={({ target }) => {
setOtp(target.value);
}}
/>
<div className="countdown-text">
{seconds > 0 || minutes > 0 ? (
<p>
Time Remaining: {minutes < 10 ? `0${minutes}` : minutes}:
{seconds < 10 ? `0${seconds}` : seconds}
</p>
) : (
<p>Didn't recieve code?</p>
)}
<button
disabled={seconds > 0 || minutes > 0}
style={{
color: seconds > 0 || minutes > 0 ? "#DFE3E8" : "#FF5630",
}}
onClick={resendOTP}
>
Resend OTP
</button>
</div>
<button className="submit-btn">SUBMIT</button>
</div>
</div>;
Handling Resend OTP
const resendOTP = () => {
setMinutes(1);
setSeconds(30);
};
When the user clicks on the Resend OTP button we invoke the resendOTP() function. Inside this function, we are resetting the minutes and seconds values and we can also make the API call to send the OTP to the user here.
Also, check out:
Output
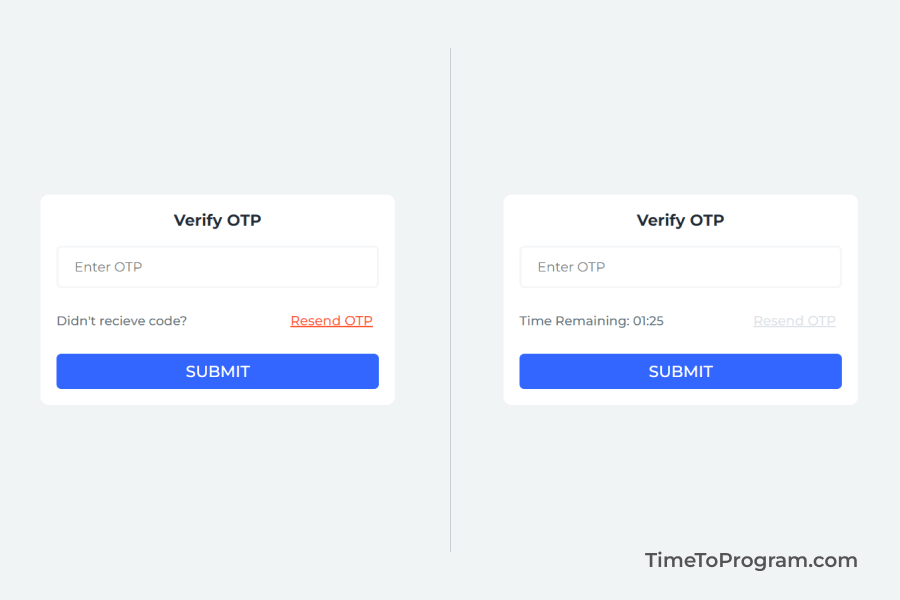