In today’s tutorial, we will learn how to create an automatic popup window using HTML CSS, and Javascript. The popup windows will be useful to display some important information or alert the user on something.
The automatic popups can be shown immediately after the web page is loaded. We see this kind of automatic popup form like email newsletter form, feedback form, etc on some websites.
Implementing this kind of automatic popups is simple. Here is the demo video of our popup which we are going to create in this tutorial.
As you can see in this above demo video, we have our simple automatic popup window which will be shown after the web page is completely loaded. And we have also added 3 seconds delay before showing the popup. Also, we have added slide-in and slide-out animation to our popup.
For hiding the popup window we have a close icon button on the top right corner. The popup window will be hidden when the user clicks on this close button.
Steps to Create Automatic Popup Window using HTML CSS and Javascript
Let’s first create the popup window UI using HTML CSS. Then we will use some javascript to show and hide the popup window.
Create Popup Window UI using HTML
<div class="popup-card">
<button id="close-btn">
<span class="material-symbols-rounded close-icon"> close </span>
</button>
<div>
<span class="material-symbols-rounded icon"> forum </span>
</div>
<div class="content">
<h3>Welcome Back!</h3>
<p>
This is a custom Automatic Popup window. You can close this popup by
clicking on the close icon on top right corner.
</p>
</div>
</div>
In the above HTML code, we have added an <div> element with the class name `popup-card` inside that we have our popup title, description, and close button. As you can see we have used google material symbols for icons.
Style The Popup Window using CSS
styles.css
body {
width: 100vw;
height: 100vh;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
background-color: #FF1744;
}
.popup-card {
width: 22rem;
display: flex;
align-items: flex-start;
gap: 1rem;
background-color: #ffffff;
border-radius: 0.2rem;
padding: 2rem 1.5rem;
position: relative;
transition: all 0.8s ease;
transform: translateY(-70vh);
}
.icon {
width: 2.8rem;
height: 2.8rem;
display: flex;
align-items: center;
justify-content: center;
background-color: #FFDDDE;
color: #e6153d;
border-radius: 50%;
}
.content > h3 {
font-size: 1.1rem;
font-weight: 600;
color: #404656;
}
.content > p {
font-size: 0.8rem;
font-weight: 500;
line-height: 1.2rem;
color: #666b78;
margin-top: 0.5rem;
}
button {
width: 1.5rem;
height: 1.5rem;
display: flex;
align-items: center;
justify-content: center;
color: #ffffff;
background-color: #e6153d;
cursor: pointer;
border: none;
border-radius: 50%;
position: absolute;
right: 1rem;
top: 1rem;
}
.close-icon {
font-size: 1rem;
}
We have added some styles to the popup-card class to make it look like a popup window and we have moved our popup window using the transform: translateY(-70vh);
property so that our popup won’t be visible when the browser window loads.
And we have added transition: all 0.8s ease;
to create a slide-in and slide-out animation effect when the popup is shown and hidden.
We have also styled our close button and placed it top right corner of the popup window using the position property. After adding these styles our popup card will look like this.
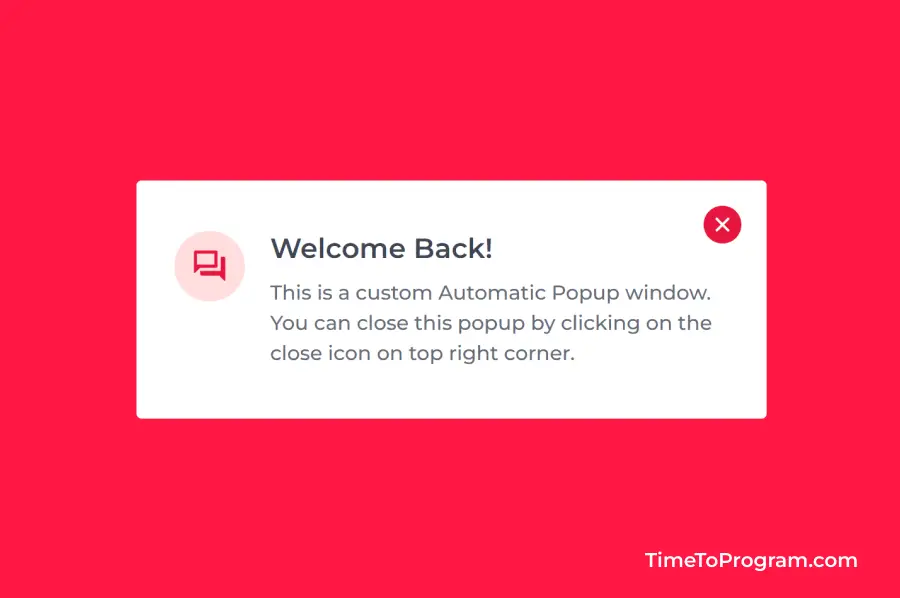
Automatically Open the Popup Window using Javascript
script.js
let popup = document.getElementsByClassName("popup-card")[0];
let closeBtn = document.getElementById("close-btn");
function showPopup() {
setTimeout(() => {
popup.style.transform = "translateY(0)";
}, 3000);
}
closeBtn.addEventListener("click", (e) => {
popup.style.transform = "translateY(-70vh)";
});
window.onload = showPopup();
In the above Javascript code, we are first getting the popup and the close button element using javascript. Then we have written the showPopup() function to show the popup window with a delay of 3 seconds.
The showPopup() function will be invoked after the webpage is loaded completely.
For adding the delay we have used the setTimeout() method. Inside setTimeout(), we are updating the popup-card transform property value to translateY(0)
. This will bring the popup window inside the visible viewport.
Inside the close button event listener, we have set the popup-card transform property value back to translateY(-70vh)
. This will again move the popup window outside the viewport’s visible area.
Also check out:
Conclusion
That’s it, we have created a simple and beautiful automatic popup window using HTML CSS, and Javascript. And we have also added a slide animation effect to it. Hope it was helpful.