Today in this article, we will learn how to pass data from one component (parent component) to another (child component) in React JS.
It is essential to pass data from one component to another in a single-page application so that communication between the components happens. In react, we pass data using props. Props can be passed to a component like an attribute that we use in HTML.
Let’s understand with an example, how to pass data from the parent component to the child component. In this example, we are going to use react function component.
Pass data from parent to child component react | Demo Video
Firstly we have created a new project using create-react-app. Then have to create two components which are the parent and child components. Let’s consider App.js as a parent component.
App.js
import logo from "./logo.svg";
import "./App.css";
import { useState } from "react";
import ChildComponent from "./ChildComponent";
function App() {
const [message, setMessage] = useState("");
return (
<div className="App-container">
<div className="card">
<div className="card-header">
<h4 className="title">Parent Component</h4>
</div>
<div className="card-body">
<p className="label">Message</p>
<input
type="text"
value={message}
onChange={({ target }) => {
setMessage(target.value);
}}
placeholder='Enter Message'
/>
</div>
</div>
<ChildComponent messageString={message} />
</div>
);
}
export default App;
In App.js, we have an input element and we have created a state (message) using the useState hook to manage the input value. Then we will import the ChildComponent and pass the input value (message) to the child.
ChildComponent.js
import React from 'react'
function ChildComponent(props) {
return (
<div className="card">
<div className="card-header">
<h4 className="title">Child Component</h4>
</div>
<div className="card-body">
<p className="label">Your Message</p>
<p className='text'>{props.messageString}</p>
</div>
</div>
)
}
export default ChildComponent
In the child component, we are accessing the props and displaying the input value.
Also check out:
Output
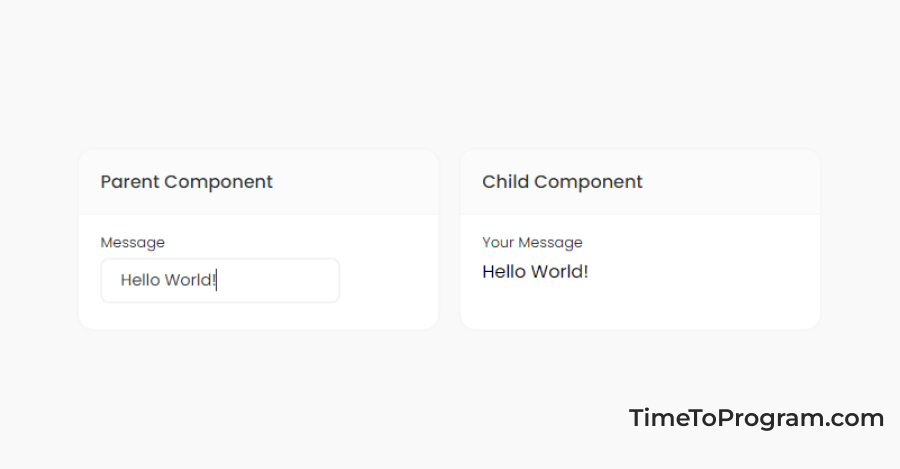