Today in this blog post, we will learn how to play sound when a user clicks on a button using HTML and Javascript. To play sound or audio we can use the HTML <audio> element.
We can pass one or more audio sources to the HTML <audio> element and the browser will select the appropriate audio file. Below is the example code for using <audio>.
<!-- One Audio Source -->
<audio controls src="./audio.mp3"></audio>
<!-- Multiple Audio Source -->
<audio controls>
<source src="./audio.mp3" type="audio/mpeg" />
<source src="./audio2.ogg" type="audio/ogg" />
</audio>
In this tutorial instead of using <audio> HTML element, we will be using the Audio() constructor to create the audio HTML element and using that we play and pause audio in javascript.
Play Sound on Button Click in HTML Javascript
As shown in the above demo video, we have created a play and pause button using HTML. When the user clicks on the play button we play the audio file using javascript. Similarly, when the pause button is clicked we stop playing the audio.
Step1: Create Play and Pause Button in HTML
<p>Play Audio</p>
<div class="action-btns">
<button class="btn play">Play</button>
<button class="btn pause">Pause</button>
</div>
In the above HTML code, we have added two buttons one for playing the audio and another button to pause the currently playing audio. Used some CSS to style our buttons.
styles.css
* {
margin: 0px;
padding: 0px;
}
body {
width: 100vw;
height: 100vh;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
background-color: #EBFC75;
}
p {
font-size: 1.5rem;
font-weight: 600;
text-align: center;
color: #17193F;
margin-bottom: 20px;
}
.action-btns {
display: flex;
align-items: center;
gap: 2rem;
}
.btn {
width: 12rem;
font-size: 1rem;
font-weight: 600;
color: #ffffff;
border: none;
border-radius: 0.2rem;
padding: 0.5rem;
cursor: pointer;
}
.play {
background-color: #89B016;
}
.play:hover{
background-color: #6e8d12;
}
.pause {
background-color: #E7CF20;
}
.pause:hover{
background-color: #d0ba1d;
}
Step2: Play Sound When Button Clicked in Javascript
script.js
let playBtn = document.getElementsByClassName("play");
let pauseBtn = document.getElementsByClassName("pause");
let text = document.querySelector("p");
const audio = new Audio("./audio.mp3");
playBtn[0].addEventListener("click", (e) => {
audio.play();
text.innerHTML = "Audio Playing🔊";
});
pauseBtn[0].addEventListener("click", (e) => {
audio.pause();
text.innerHTML = "Audio Paused";
});
Here we have used the Audio() constructor to create a new HTML audio element and we are passing the audio file path as a parameter to it. Then added event listeners for both the play and pause buttons.
Inside the event listener of the play button, we are using audio.play() method to play the audio file and we are updating the <p> tag text to `Audio Playing 🔊`.
Similarly, inside the pause button event listener, we are using audio.pause() method to pause the playing audio. And updating the <p> tag innerHTML to `Audio Paused`.
Also check out:
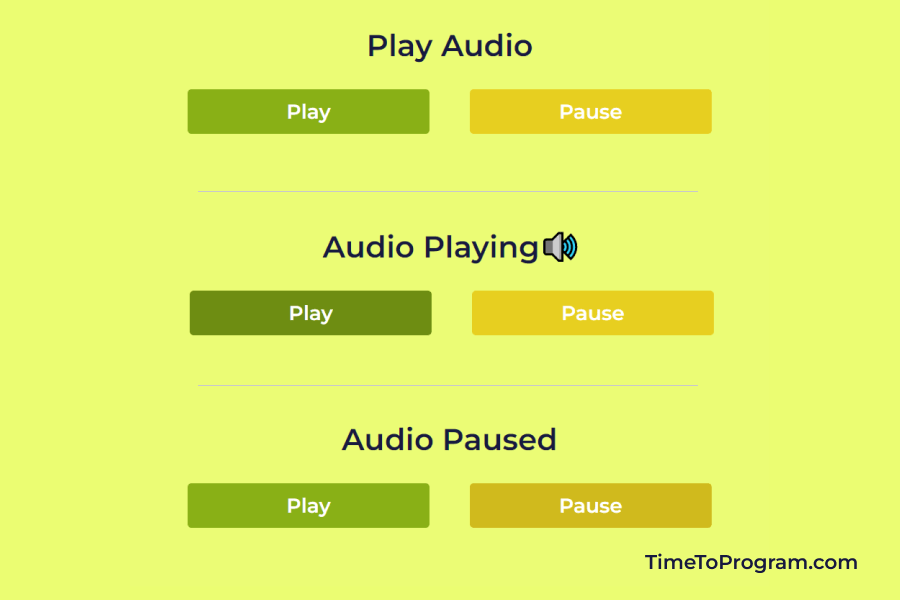
Conclusion
In this short tutorial, we have learned how to play and pause sound or audio on a button click using HTML and Javascript. Hope it was helpful.