In this post, we will learn how to add weeks to the current date in Javascript. Sometimes in our projects, we come across some situations where we want to add weeks to a given date or current date. We can do it using a single line of code in javascript.
To get the number of weeks and the current date from the user let’s first create a simple form using HTML and CSS. Then we will add the number of weeks entered by the user to the current or given date. Here is the demo video to understand better.
Create HMTL Form to Get the Number of Weeks and Date
<div class="card">
<h3>Add Weeks to Current Date</h3>
<form>
<input
type="number"
name="weeks"
id="weeks"
placeholder="Enter Number of Weeks"
/>
<input
type="date"
name="currentDate"
id="currentDate"
placeholder="Select Current Date"
/>
<input type="submit" id="submit-btn" />
</form>
<label>Output:</label>
<p id="result">--/--/--</p>
</div>
styles.css
body {
width: 100vw;
height: 100vh;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
background-color: #32b4b3;
}
.card {
width: 25rem;
gap: 1rem;
background-color: #ffffff;
border-radius: 0.2rem;
padding: 2rem 1.5rem;
}
h3 {
font-size: 1.1rem;
font-weight: 700;
color: #404656;
margin-bottom: 1rem;
}
input {
width: 23rem;
border: none;
border-radius: 0.2rem;
background-color: #eaf7f8;
padding: 0.8rem 1rem;
margin-bottom: 1rem;
}
input:focus {
outline: none;
}
input[type="submit"] {
width: 100%;
font-size: 0.9rem;
font-weight: 500;
border: none;
border-radius: 0.2rem;
color: #ffffff;
background-color: #32b4b3;
padding: 0.8rem 1rem;
cursor: pointer;
}
input[type="submit"]:hover {
color: #32b4b3;
background-color: #d6f0f0;
}
label {
font-size: 0.9rem;
font-weight: 600;
}
p {
font-size: 0.8rem;
font-weight: 500;
margin-top: 0.5rem;
}
In the above code, we have written the HTML code to create a form that contains the input type of `number` to enter the number of weeks, the input type `date` to select the date, and a submit button. Also Added some CSS styles to our form to make it look good.
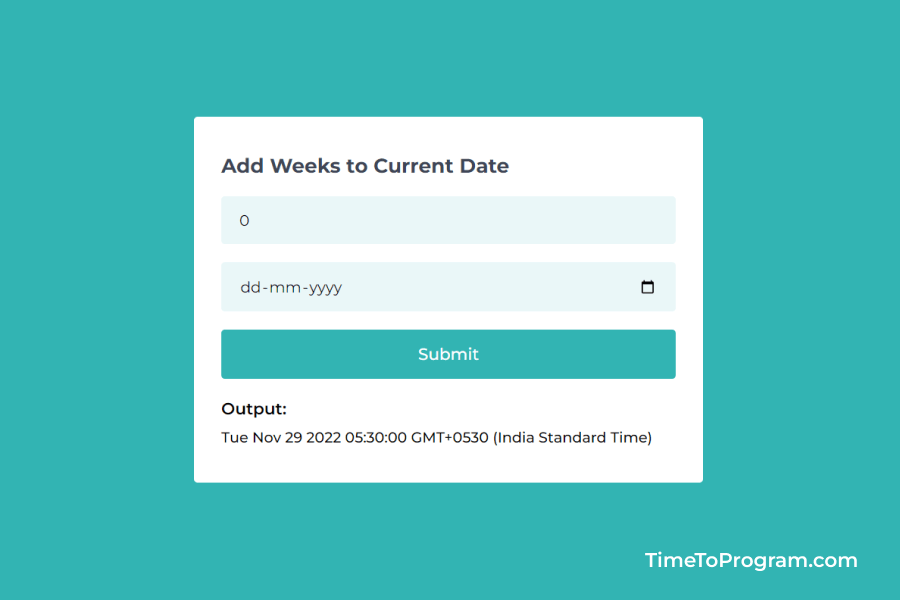
Javascript Code to Add Weeks to Given Date
script.js
let weeks = document.getElementById("weeks");
let date = document.getElementById("currentDate");
let submitBtn = document.getElementById("submit-btn");
let result = document.getElementById("result");
submitBtn.addEventListener("click", (e) => {
e.preventDefault()
const numberOfWeeks = weeks.value;
const currentDate = new Date(date.value);
currentDate.setDate(currentDate.getDate() + numberOfWeeks * 7)
result.innerHTML = currentDate
});
In the above code, we have written the logic to add weeks to a given date inside the submit button event listener. First, we are getting the weeks values and user-given date.
For adding weeks to date, we use the ‘date.setDate()’ method. Basically, to add a single week to the current date we need to add seven days to our current date. For multiple weeks we will multiply the number of weeks by 7 and we add that to our current date.
After adding the weeks to date we will be displaying the result on the screen.
Function to Add Weeks to Date Using Javascript
Here I have written a separate javascript function to add weeks to a specific date. We can use this function in our projects.
function addWeeksToDate(numberOfWeeks, currentDate) {
currentDate.setDate(currentDate.getDate() + numberOfWeeks * 7)
return currentDate
}
const result = addWeeksToDate(2, new Date())
console.log(result);
// Output
// Tue Dec 13 2022 09:43:30 GMT+0530 (India Standard Time)
Here we have written the ‘addWeeksToDate’ function, it will take the number of weeks and date as an argument. Inside this function, we are adding the number of weeks to the given date using the ‘date.setDate()’ method and return the result.
Also check out: