In this blog post, we are going to learn how to save Textarea value to a file using HTML and Javascript. In our projects, we may come across some situations where we want to store the text entered by the user inside a file programmatically.
Today we are going the build a simple javascript project in which we will store the text entered by the user into a file programmatically. And after saving the file will be downloaded. Here is the demo video of our project.
As you can see in the above demo video, we have created a simple HTML form with text area input to enter the text, an input box to enter the filename, and a Save & Download button.
When the user clicks on the save and download button, we will save the content of the textarea into a file. We name the file with user entered file name and the file will be downloaded.
How to Save Textarea/Input Value to Text File in Javascript
To begin with, let’s first create a simple form using HTML and CSS to enter the text data and file name. Then we will save the text data into a file and download using javascript.
Create HTML Form To Enter Text and File Name
<div class="form-card">
<h3>Save Text to File</h3>
<form>
<textarea id="text" placeholder="Enter Text"></textarea>
<input type="text" id="fileName" placeholder="Enter File Name" />
<input type="submit" value="Save & Download" id="saveBtn" />
</form>
</div>
In the above HTML code, we have added an <textarea>
element to enter the text, added input of type `text` to enter the file name, and added an input submit button with the value `Save & Download`. Now, let’s style this form using CSS.
styles.css
body {
width: 100vw;
height: 100vh;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
background-color: #093666;
}
.form-card {
width: 25rem;
gap: 1rem;
background-color: #ffffff;
border-radius: 0.3rem;
padding: 1.5rem;
}
h3 {
font-size: 1.1rem;
font-weight: 700;
color: #404656;
margin-bottom: 1rem;
}
input[type='text'], textarea {
width: 23rem;
border: none;
border-radius: 0.2rem;
background-color: #e6ebf0;
padding: 0.8rem 1rem;
margin-bottom: 1rem;
}
input[type='submit'] {
width: 100%;
font-size: 0.9rem;
font-weight: 600;
border: none;
border-radius: 0.2rem;
color: #ffffff;
background-color: #062647;
padding: 0.8rem 1rem;
cursor: pointer;
}
input[type='submit']:hover{
color: #ffffff;
background-color: #093666;
}
Here we have some styles to textarea
, input[type=’text’]
, and input[type=’submit’]
. After adding these styles our form looks something like this.
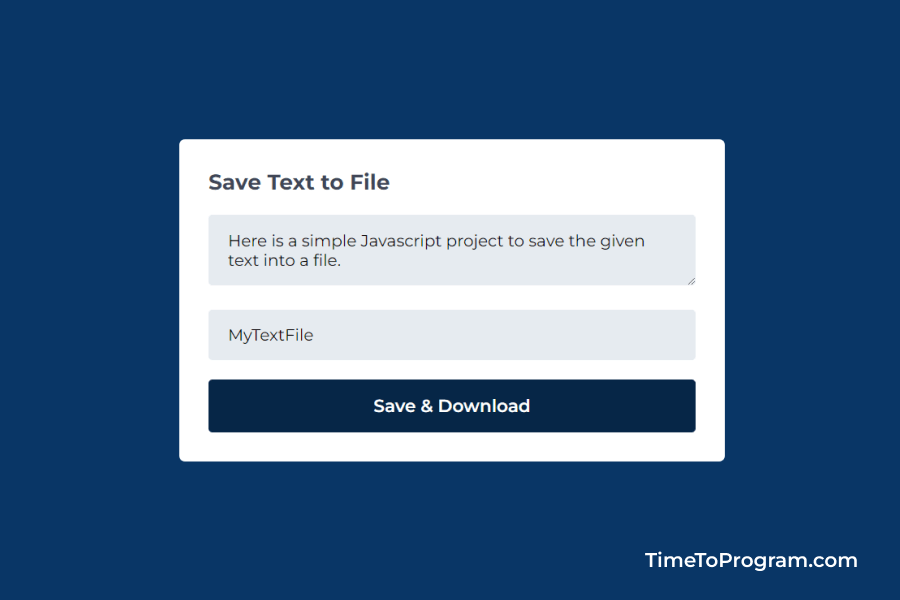
Javascript Code to Save Text into File
script.js
let textEle = document.getElementById("text");
let fileNameEle = document.getElementById("fileName");
let saveBtn = document.getElementById("saveBtn");
saveBtn.addEventListener("click", (e) => {
e.preventDefault();
const textData = textEle.value;
const textDataBlob = new Blob([textEle.value], { type: "text/plain" });
const downloadUrl = window.URL.createObjectURL(textDataBlob)
const downloadLink = document.createElement('a');
downloadLink.download = fileNameEle.value
downloadLink.href = downloadUrl;
downloadLink.click()
console.log(textData);
console.log(textDataBlob);
});
In the above javascript code, we have a click event listener to the save button. Inside that, we have written the logic for saving the text into a file and downloading the file.
Inside the saveBtn event listener, we are first getting the text data and filename entered by the user. Then we are constructing the blob using the new Blob(). The Blob() constructor is used to create a blob from other objects like JSON, text, etc. You can learn more above blob here.
After creating the Blob, we created the file URL using URL.createObjectUrl() static method. Then created an anchor element using the `createElement()
` method and added the newly created file URL to the href attribute. Finally, we are calling the `click()
` method on the anchor element to start the file downloading.
Also check out:
Conclusion
In this tutorial, we have learned how to save textarea/ input value to a text file using javascript. Hope it was helpful. Thanks