In this post, we will look at how to copy text to the clipboard in React JS with an example. We are going to implement copy text to clipboard using a library called react-copy-to-clipboard and will also implement it without using any library.
Copy text to clipboard using react-copy-to-clipboard
1. Installing the react-copy-to-clipboard library
Let’s first install the react-copy-to-clipboard package using this command.
npm i react-copy-to-clipboard
2. Using the library
Now we are going to create the UI for this example. Our UI contains a simple card with a textarea input and a copy button.
For implementing copy text to clipboard functionality. We will use the CopyToClipboard component that we get from the react-copy-to-clipboard library.
import "./App.css";
import { useState } from "react";
import { CopyToClipboard } from "react-copy-to-clipboard";
function App() {
const [text, setText] = useState("Copy this text to clipboard");
const [isCopied, setIsCopied] = useState(false);
return (
<div className="App-container">
<div className="card">
<div className="card-header">
<h4 className="title">Copy Text to Clipboard</h4>
</div>
<div className="card-body">
<p className="label">Message</p>
<textarea
type="text"
value={text}
onChange={({ target }) => {
setText(target.value);
}}
placeholder="Enter Message"
className="text-area"
/>
{isCopied ? (
<p className="success-msg">Text copied to clipboard</p>
) : null}
<CopyToClipboard
text={text}
onCopy={() => {
setIsCopied(true);
setTimeout(() => {
setIsCopied(false);
}, 1000);
}}
>
<button className="btn">COPY</button>
</CopyToClipboard>
</div>
</div>
<textarea
type="text"
className="text-area"
style={{ marginTop: "10px" }}
/>
</div>
);
}
export default App;
We have placed our copy button in between the opening and closing tag of the CopyToClipboard component. Now we are passing the text that we need to copy to the clipboard to the CopyToClipboard component.
Now when the user clicks on the copy button, the text which we passed to the CopyToClipboard component will be copied to the clipboard.
After the text is copied, we show a success message “Text copied to clipboard” for 1 second.
3. Output
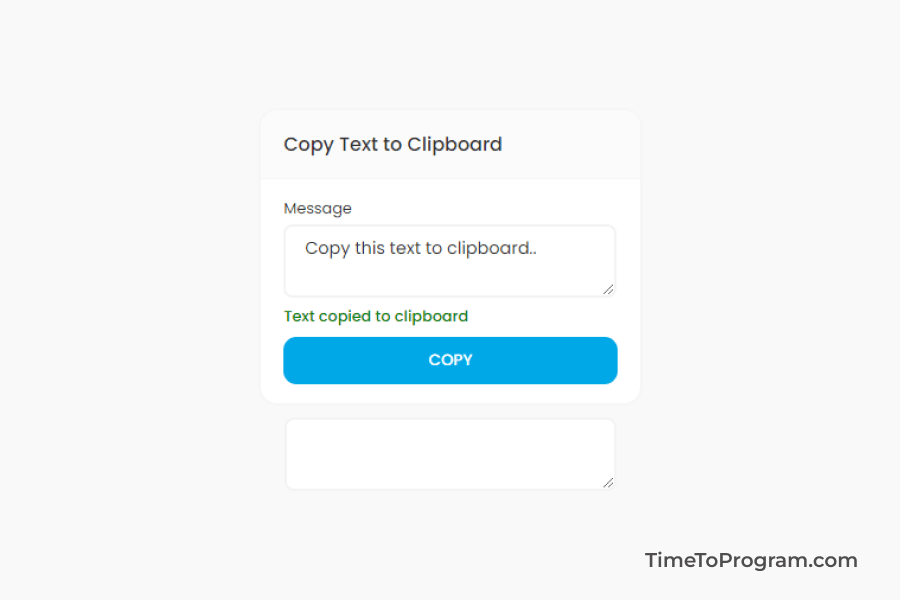
Copy text to the clipboard using navigator
To copy text to the clipboard without using any packages. We are going to use the navigator object in javascript. That allows us to access the system clipboard and do some operations like copy, paste, and cut.
import "./App.css";
import { useState } from "react";
function App() {
const [text, setText] = useState("Copy this text to clipboard");
const [isCopied, setIsCopied] = useState(false);
const handleClick = () => {
navigator.clipboard.writeText(text);
setIsCopied(true);
setTimeout(() => {
setIsCopied(false);
}, 1000);
};
return (
<div className="App-container">
<div className="card">
<div className="card-header">
<h4 className="title">Copy Text to Clipboard</h4>
</div>
<div className="card-body">
<p className="label">Message</p>
<textarea
type="text"
value={text}
onChange={({ target }) => {
setText(target.value);
}}
placeholder="Enter Message"
className="text-area"
/>
{isCopied ? (
<p className="success-msg">Text copied to clipboard</p>
) : null}
<button onClick={handleClick} className="btn">
COPY
</button>
</div>
</div>
<textarea
type="text"
className="text-area"
style={{ marginTop: "10px" }}
/>
</div>
);
}
export default App;
Here inside the handleClick function, we are using the ‘navigator.clipboard.writeText()’ method to copy the text. This method takes the text that needs to be copied as a parameter.
Conclusion
These are the two ways to copy text to the clipboard. Hope this post helped you.
Also check out: