Today in this blog post, we will create a simple like and dislike button in React JS. In this example, we will also display the likes and dislikes count inside the button so that when the user clicks on the like or dislike button we will change the likes and dislikes count.
Steps to Create Like and Dislike Button in React JS
- Declaring React state variables
- Creating the like and dislike button
- Handling the like and dislike button click
Declaring React state variables
Let’s first declare some React useState variables to hold the likes and dislikes count.
const [likeCount, setLikeCount] = useState(50);
const [dislikeCount, setDislikeCount] = useState(25);
const [activeBtn, setActiveBtn] = useState("none");
Here we have also defined a state variable ‘activeBtn’ to store the active button state.
Also Checkout:
Creating the like and dislike button
Now we will write the JSX code to create two buttons and we will also display the likes and dislikes count inside our buttons.
App.js
<div className="container">
<div className="btn-container">
<button
className={`btn ${activeBtn === "like" ? "like-active" : ""}`}
onClick={handleLikeClick}
>
<span className="material-symbols-rounded">thumb_up</span>
Like {likeCount}
</button>
<button
className={`btn ${activeBtn === "dislike" ? "dislike-active" : ""}`}
onClick={handleDisikeClick}
>
<span className="material-symbols-rounded">thumb_down</span>
Dislike {dislikeCount}
</button>
</div>
</div>;
index.css
* {
font-family: "Poppins", sans-serif;
padding: 0;
margin: 0;
}
.container {
width: 100vw;
height: 100vh;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
background-color: #f0f1f3;
}
.btn-container {
display: flex;
flex-direction: 'row';
align-items: center;
background-color: #fff;
padding: 30px 50px;
border-radius: 12px;
}
.btn {
border: none;
color:#fff;
font-size: 0.8rem;
display: flex;
align-items: center;
gap:6px;
background-color: #bdc0c5;
border-radius: 4px;
padding: 6px 15px;
margin: 0 10px;
}
.like-active {
background-color: #FF0063;
}
.dislike-active {
background-color: #394350;
}
For displaying the like and dislike icons, we are using material symbols. In the above JSX code, we are adding the ‘like-active’ and ‘dislike-active’ CSS class to the like and dislike button based on the ‘activeBtn’ status.
Handling the like and dislike button click
To handle the button clicks, we have written the ‘handleLikeClick’ function to handle like button clicks and for handling dislike button clicks we have written the ‘handleDislikeClick’ function.
const handleLikeClick = () => {
if (activeBtn === "none") {
setLikeCount(likeCount + 1);
setActiveBtn("like");
return;
}
if (activeBtn === 'like'){
setLikeCount(likeCount - 1);
setActiveBtn("none");
return;
}
if (activeBtn === "dislike") {
setLikeCount(likeCount + 1);
setDislikeCount(dislikeCount - 1);
setActiveBtn("like");
}
};
Inside the ‘handleLikeClick’ function, we are checking if the ‘activeBtn’ value is equal to ‘none’. If true, we are incrementing ‘likeCount’ by one and setting the ‘activeBtn’ value to ‘like’.
If the ‘activeBtn’ value is equal to ‘like’ then the user is already clicked the like button. so we need to decrease the ‘likeCount’ by 1 and set the ‘activeBtn’ value to ‘none’.
Finally, if the ‘activeBtn’ value is equal to ‘dislike’, we will increment ‘likeCount’ by 1, decrease ‘dislikeCount’ by 1, and set the ‘activeBtn’ value to ‘like’.
Here is the code for the ‘handleDislikeClick’ function.
const handleDisikeClick = () => {
if (activeBtn === "none") {
setDislikeCount(dislikeCount + 1);
setActiveBtn("dislike");
return;
}
if (activeBtn === 'dislike'){
setDislikeCount(dislikeCount - 1);
setActiveBtn("none");
return;
}
if (activeBtn === "like") {
setDislikeCount(dislikeCount + 1);
setLikeCount(likeCount - 1);
setActiveBtn("dislike");
}
};
The ‘handleDislikeClick’ function will work just like the opposite of the ‘handleDislikeClick’ function.
Output
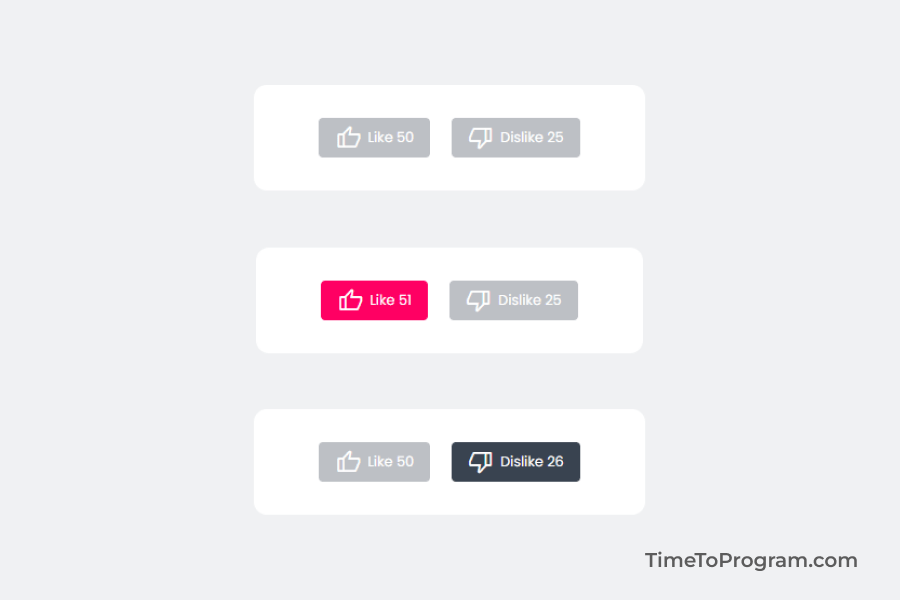