Today in this blog post, we will create a simple horizontal drag and drop list in React JS. we have already written a blog post discussing how to implement a vertical drag and drop list. You can check out that post here.
In this example, we will create a horizontal list where users can drag and drop the list items inside the container. We will use the react-beautiful-dnd library to implement a horizontal drag and drop list.
Steps to Create Horizontal Drag and Drop List in React
- Install react-beautiful-dnd library
- Create JSX code for horizontal drag and drop list
- Update the list item position after each drag
1. Install the react-beautiful-dnd library
Let’s install the react-beautiful-dnd package using this command.
npm i react-beautiful-dnd
2. Create JSX code for horizontal drag and drop list
<div className="container">
<div className="card">
<div className="header">Horizontal Drag and Drop List</div>
<DragDropContext onDragEnd={onDragComplete}>
<Droppable droppableId="drag-drop-list" direction="horizontal">
{(provided, snapshot) => (
<div
className="drag-drop-list-container"
{...provided.droppableProps}
ref={provided.innerRef}
>
{dragDropList.map((item, index) => (
<Draggable key={item.id} draggableId={item.label} index={index}>
{(provided) => (
<div
className="item-card"
ref={provided.innerRef}
{...provided.draggableProps}
{...provided.dragHandleProps}
>
<span className="material-symbols-outlined">
drag_indicator
</span>
<div className="char-avatar">{item.label.charAt(0)}</div>
<p className="label">{item.label}</p>
</div>
)}
</Draggable>
))}
{provided.placeholder}
</div>
)}
</Droppable>
</DragDropContext>
</div>
</div>;
Here we have written the JSX code to implement the horizontal drag and drop list.
First, we have defined a constant variable ‘listItems’ to store the list of items. And we have set this list data to a state variable ‘dragDropList’.
We are using the DragDropContext, Droppable, and Draggable from the react-beautiful-dnd library to implement the drag and drop list.
To make horizontal drag and drop work, we need to set the ‘direction’ prop as ‘horizontal’ on the <Droppable /> component.
3. Update the list item position after each drag
Finally, we will update our list items whenever the drag is complete. To do that we have written the onDragComplete function.
const onDragComplete = (result) => {
if (!result.destination) return;
const arr = [...dragDropList];
//Changing the position of Array element
let removedItem = arr.splice(result.source.index, 1)[0];
arr.splice(result.destination.index, 0, removedItem);
//Updating the list
setDragDropList(arr);
};
In this function, we check whether the item has been dropped outside of the container or not. If true, we are not going to anything. If the list item is dropped within the container, we will update the item position using the Array.splice() method.
And we set the updated list to our ‘dragDropList’ state variable.
Also check out:
- Verticle drag and drop list example in React
- Create a checkbox list in React
- How To Use Session Storage in React
Output
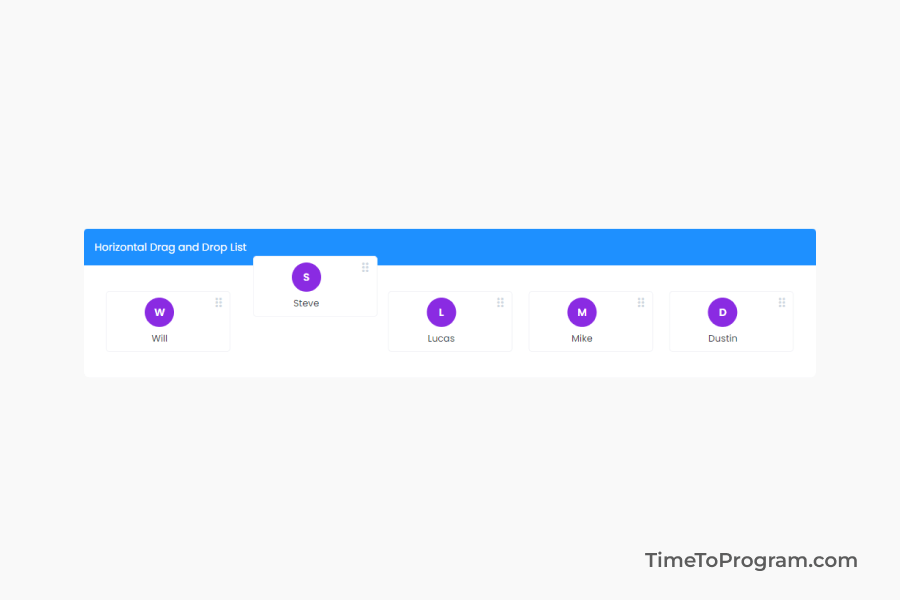